Unlocking the Power of Data: A Beginner's Guide to PySpark for Big Data Analytics
- dataUology
- Apr 21, 2024
- 2 min read
Updated: Apr 29, 2024
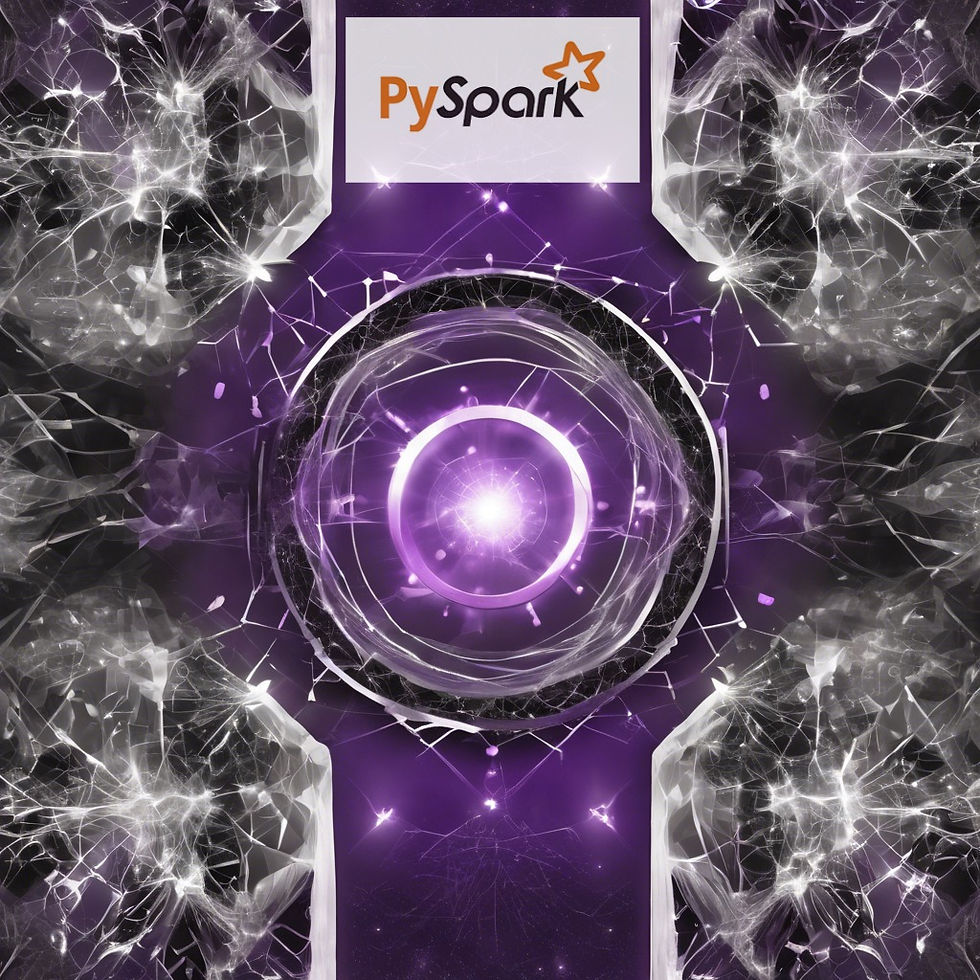
In today's data-driven world, PySpark has emerged as a powerhouse, seamlessly blending Python's simplicity with Apache Spark's distributed computing engine. This guide is your roadmap to mastering PySpark, from its fundamental concepts to advanced analytics techniques, equipping you to extract valuable insights from your data with ease.
Understanding Apache PySpark
What is PySpark?
PySpark serves as the Python API for Apache Spark, offering a bridge between Python and Spark's distributed computing capabilities. It enables users to leverage Spark's speed and scalability without leaving the familiar Python environment.
Key Features
PySpark boasts a rich set of features, including its DataFrame API for structured data processing, support for SQL queries, machine learning algorithms via MLlib, and graph processing capabilities with GraphFrames.
Getting Started with PySpark
Installation and Setup
Installing PySpark
Begin by installing PySpark on your local machine or cluster environment. For instance, to install PySpark locally via pip, you can use the command: `pip install pyspark`.
Configuration
Configure PySpark to connect to your Spark cluster by setting environment variables like `SPARK_HOME` and `PYTHONPATH`.
Basic Operations
Loading Data
Load data into PySpark from various sources such as CSV files, JSON files, databases, or HDFS. For example, to load a CSV file into a DataFrame, you can use: `df = spark.read.csv("data.csv", header=True)`.
Data Manipulation
Perform basic data manipulation tasks using PySpark's DataFrame API. For instance, you can filter rows based on a condition: `filtered_df = df.filter(df["column"] > 100)`.
Advanced Analytics with PySpark
PySpark for Machine Learning
Building Models
Utilize PySpark's MLlib library to build machine learning models. For example, to train a linear regression model, you can use: `from pyspark.ml.regression import LinearRegression` followed by model training and evaluation steps.
Model Evaluation
Evaluate model performance using techniques like cross-validation and hyperparameter tuning. For instance, you can perform cross-validation using the `CrossValidator` class in PySpark.
PySpark for Graph Processing
Graph Analysis
Analyze large-scale graphs using PySpark's graph processing capabilities. You can calculate centrality measures or detect communities in a graph using GraphFrames.
Social Network Analysis
Apply PySpark to analyze social networks. For example, you can identify influencers in a social network by analyzing the network structure and node properties.
Simple Ways To Optimization
Data Partitioning
Optimize data partitioning to improve performance in PySpark. For example, you can repartition a DataFrame based on a specific column to achieve better parallelism.
Caching and Persistence
Cache intermediate results or persist data in memory or disk for faster access. Use `cache()` or `persist()` methods on DataFrames to achieve this.
Real-World Applications of PySpark
E-commerce Analytics
Employ PySpark for e-commerce analytics tasks such as customer segmentation, recommendation systems, and sales forecasting.
Cybersecurity Analytics
Leverage PySpark for cybersecurity analytics, including network traffic analysis, anomaly detection, and threat identification.
Conclusion
With PySpark, the possibilities for big data analytics are endless. By mastering PySpark's features and techniques outlined in this guide, you can unlock the full potential of your data, driving innovation and gaining a competitive edge in today's data-driven landscape. Start your PySpark journey today and transform your big data analytics workflows like never before.
In some later posts, I will show examples of using PySpark and SparkR